iOS7 requires new images for the icons and launch image and Xcode now provides a new way of validating that you have all of the required images.
Just to see what Xcode would do, I created a new app from scratch and looked at the images it requires.
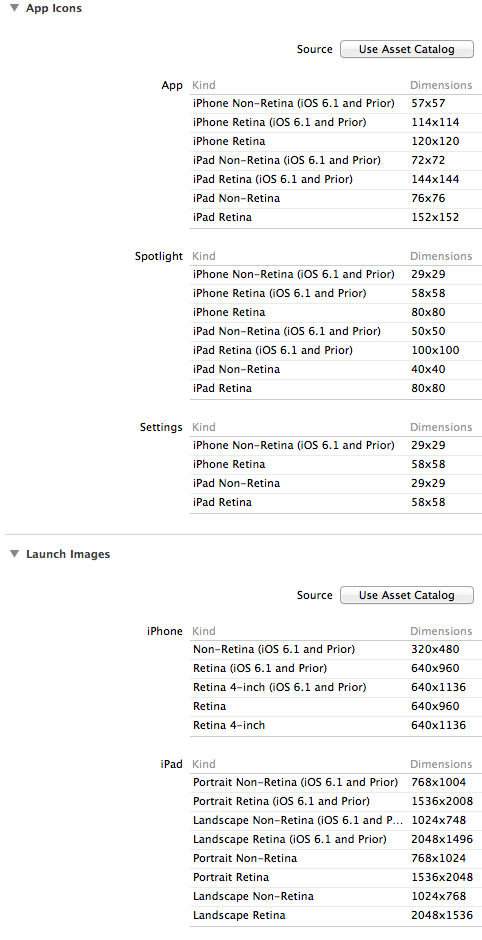
I then took a look at what an existing app looks like. The first thing I noticed is that I forgot to include the 100×100 iPad retina image. Then I noticed that the last four launch images are the same as the ones that I already have.
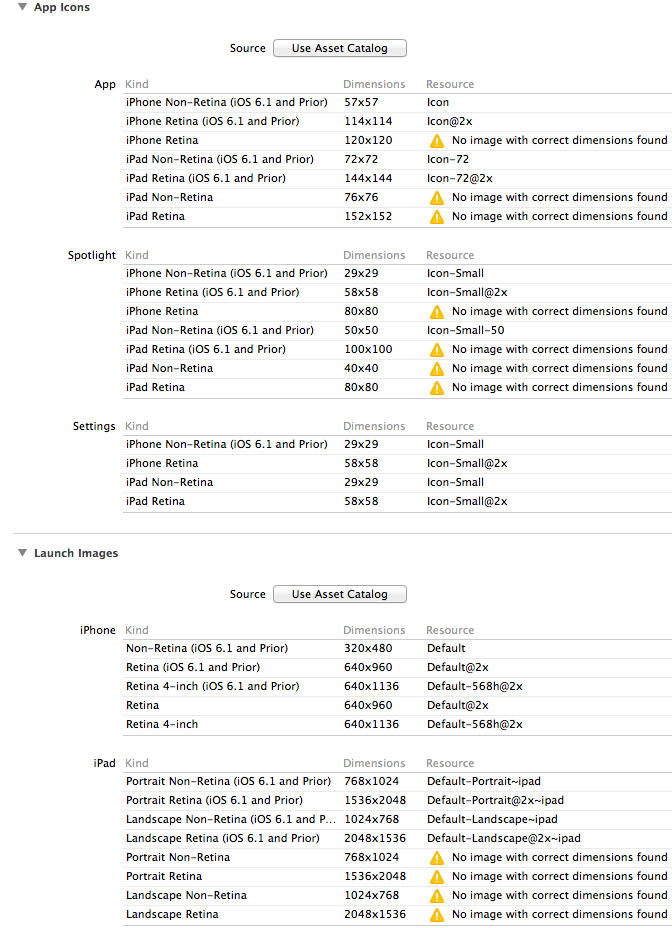
I manually added the Portrait Non-Retina and it automatically found the retina version. Then I added the Landscape Non-Retina and it found the retina. I looked in the .plist for the app and Xcode had added lines for the new files. Since I have over 20 apps I didn’t want to repeat this 20 or more times so I right-clicked on the file, opened the .plist as source, then copied the following lines.
<key>UILaunchImages~ipad</key>
<array>
<dict>
<key>UILaunchImageMinimumOSVersion</key>
<string>7.0</string>
<key>UILaunchImageName</key>
<string>Default-Landscape</string>
<key>UILaunchImageOrientation</key>
<string>Landscape</string>
<key>UILaunchImageSize</key>
<string>{768, 1024}</string>
</dict>
<dict>
<key>UILaunchImageMinimumOSVersion</key>
<string>7.0</string>
<key>UILaunchImageName</key>
<string>Default-Portrait</string>
<key>UILaunchImageOrientation</key>
<string>Portrait</string>
<key>UILaunchImageSize</key>
<string>{768, 1024}</string>
</dict>
</array>
I pasted these lines into the same place in each of the .plists.
While I was at it, I added a line to my pre-iOS7 images list for the file I forgot to include. That section now looks like this:
<key>CFBundleIconFiles</key>
<array>
<string>Icon.png</string>
<string>Icon@2x.png</string>
<string>Icon-72.png</string>
<string>Icon-72@2x.png</string>
<string>Icon-Small-50.png</string>
<string>Icon-Small-50@2x.png</string>
<string>Icon-Small.png</string>
<string>Icon-Small@2x.png</string>
</array>
I then used Xcode to add the new images. (Note: As far as I can tell, you can name them anything you want. I followed a logical extension of the old rules.) I used the same image for Spotlight iPad Retina and iPhone Retina (80×80) so I name it Icon-402x.png.
Xcode added an iPad section to the .plist. The .plist now looks like this:
<key>CFBundleIconFiles</key>
<array>
<string>Icon-40</string>
<string>Icon-iPhone-60</string>
<string>Icon.png</string>
<string>Icon@2x.png</string>
<string>Icon-72.png</string>
<string>Icon-72@2x.png</string>
<string>Icon-Small-50.png</string>
<string>Icon-Small-50@2x.png</string>
<string>Icon-Small.png</string>
<string>Icon-Small@2x.png</string>
</array>
<key>CFBundleIconFiles~ipad</key>
<array>
<string>Icon-40</string>
<string>Icon-iPad-76</string>
<string>Icon-iPhone-60</string>
<string>Icon.png</string>
<string>Icon@2x.png</string>
<string>Icon-72.png</string>
<string>Icon-72@2x.png</string>
<string>Icon-Small-50.png</string>
<string>Icon-Small-50@2x.png</string>
<string>Icon-Small.png</string>
<string>Icon-Small@2x.png</string>
</array>
Xcode has a new folder type for Asset Catalogs. I did not convert my icons to asset catalogs, but it looks like a good way to manage assets.